The visual checksum is a technique to prevent the wrong code being read due to reflections or dirt.
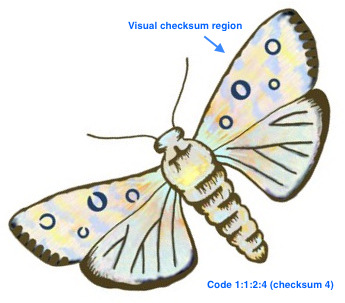
It was introduced in the paper “Enabling Hand-Crafted Visual Markers at Scale” to be presented at the ACM conference Designing Interactive Systems 2017.
Using the visual checksum is simple:
- We include a new region with a specific number of hollow blobs in our design, and
- Tell the software we’re using the visual checksum.
What are checksums?
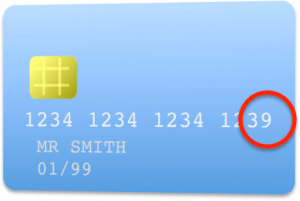
Checksums are traditionally additional numbers at the end of a list of numbers (like a credit card number) used to make sure the list of numbers has been entered, recognised or sent correctly. You use checksums everyday as the last digit of a barcode or your credit card number, it’s even used on everything you view on the internet! Checksums work by applying some mathematical formula to the list of numbers to decide what the checksum should be and the reversing the process later when we need to check everything’s correct.
The visual checksum is a visual version of this.
If the thought of math is making you reach for the back button don’t worry! The computer does all the math for you.
How do I add it to my design?
Use the box below to enter your code and it will workout the visual checksum value for you. Now just add an extra region with that many hollow blobs.
Tips
- You can only have ‘hollow blobs’ in the visual checksum region, mixing in ‘solid blobs’ will prevent it from reading.
- Make sure your ‘hollow blobs’ only have one hollow bit and don’t touch, e.g. they should look like ‘D’ rather than ‘B’.
- Like the ‘solid blobs’, the ‘hollow blobs’ can be any shape not just circles.
How do I use this in the app?
Just testing things?
If your design uses 5 regions plus the visual checksum region you can test if your new design works by opening the app and searching for “vc555” and select “Visual Checksum 5 Region Test”.
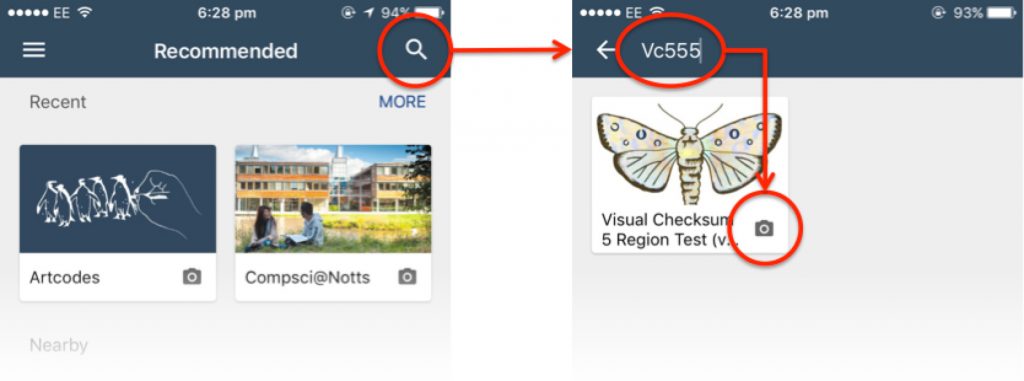
New experience
To create a new experience using the visual checksum open the Artcodes app and search for “vc123” and you will see an experience called “Visual Checksum Base”. Open this then tap “Copy” (you may be asked to sign in with your Google account if you have not already). Now tap “Edit” and edit the name, availability and codes of your experience. You’re done! Your new experience with visual checksum enabled is ready to use!
Existing experience
We are working on adding the ability to add this to existing experiences.